Welcome, Guest |
Welcome to Makestation! We are a creative arts/indie discussion community — Your center for creative arts discussion, unleashed!
Please note that you must log in to participate in discussions on the forum. If you do not have an account, you can create one here. We hope you enjoy the forum!
|
|
tc4me
December 17th, 2024
Blablablabla
|
|
tc4me
December 17th, 2024
Blablablabla
|
|
tc4me
December 17th, 2024
Blablablabla
|
|
tc4me
December 17th, 2024
Blablablabla
|
|
tc4me
December 17th, 2024
Blablablabla
|
View all updates
|
|
|
PHP - Part 4 |
Posted by: Darth-Apple - August 13th, 2013 at 4:29 PM - Forum: Software
- No Replies
|
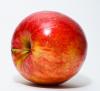 |
How to Program PHP
Tuturial, part 4
So far, we've explored several fundamental concepts in programming. We've explored the echo statement, and how a PHP file needs to be structured in order for the server to run it. In the second tutorial, an overview was given on how to use variables and arrays. In the third tutorial, functions were introduced. Functions are an extremely fundamental concept in PHP, but it can take quite a while to get used to them. If you're still a little confused, keep reading. All of this will become more clear as you continue working through these tutorials.
In this tutorial, I will introduce the concept of conditional statements. Conditional statements allow us to run different parts of our code, depending on whether a certain condition is met. For example, what if you had a script that denies registration and leaves a message for the user if the user is under 13? Many forums for example are required to deny registration to users under 13 due to COPPA restrictions in US law, so this is a popular real-world example.
Now, you have some code that echoes "sorry, but users must be at least 13 years of age to register," but you need to actually check to see if the user is at least 13 years of age before you execute that code. How exactly would you do that?
PHP has a set of "conditionals" that allow you to check for certain conditions before executing a block of code.
Code: if ($condition == 1) {
// execute this code
// this IF statement only executes the code in between the curly brackets, and it will only execute this code if the $condition variable is equal to 1.
echo "executing the IF statement code block now. ";
}
The code above won't actually do anything, but it does demonstrate that the code between the curly braces will only execute if the $condition variable is equal to one.
TIP: Notice carefully how there were two equal signs used in the IF statement for ($condition == 1). Why did we need two instead of one? Turns out = and == are different operators in PHP. if you just use =, it will try to set the $condition variable to 1 which obviously is not what we want. If you use ==, instead of trying to assign a value to the variable, it will check its existing value, which is obviously what we want. Generally, you will always use == instead of = in conditionals for PHP.
Now, the code inside of the IF statement will only execute if the $condition variable is equal to 1. What if we want to execute a separate block of code if the $condition variable IS NOT equal to 1? In this case, we'll use an IF statement, and then and ELSE statement.
Code: if ($condition == 1) {
// execute this code
// this IF statement only executes the code in between the curly brackets, and it will only execute this code if the $condition variable is equal to 1.
echo "executing the IF statement code block now. ";
}
else {
// this ELSE block will ONLY execute if the IF block does not execute. Basically, if $condition is equal to 1, PHP will execute the code inside of the IF block. If $condition is equal to anything OTHER than 1, it will execute the code in the ELSE block instead.
echo "executing ELSE block code now!";
}
As you can see, we've made use of the "else" block above. Simply put, the code in the "else" block will only run if the "if" block fails to run. If $condition is equal to anything other than 1, the else block code will run. If the $condition variable is equal to 1, the "if" block code will run. In no circumstance will both blocks of code ever run together.
So, what if it turns out that you need to execute a block of code if $condition = 1, a separate block of code if $condition = 2, and yet another separate block of code if $condition is equal to anything else? Turns out PHP has the "IF ELSE" block for that.
Code: if ($condition == 1) {
// execute this code
// this IF statement only executes the code in between the curly brackets, and it will only execute this code if the $condition variable is equal to 1.
echo "choice 1 has been selected! ";
}
if else ($condition == 2) {
// this block of code ONLY executes if the $condition variable is equal to 2.
echo "choice 2 has been selected! ";
}
else {
// this ELSE block will ONLY execute if the IF block does not execute. Basically, if $condition is equal to 1, PHP will execute the code inside of the IF block. If $condition is equal to anything OTHER than 1, it will execute the code in the ELSE block instead.
echo "Oops! We don't recognize your choice selection. An error has occurred. ";
}
As you can see, the first IF block only executes if $condition is equal to 1. The second "IF ELSE" block only executes if $condition is equal to 2. The last "ELSE" block only executes if neither of the previous two blocks of code executed. In other words, "ELSE" executes if your network of IF and IF else statements never "found a match".
There are actually other conditional statements in PHP as well. The "switch" statement is fairly popular, and there are try and catch statements as well. These aren't very important to the purpose of our tutorials and aren't essential to learning the basics of PHP, so we won't attempt to go over these in detail.
Anyway, back to the original script concept for denying user registration to users under thirteen...
Code: if ($age < 13) {
echo "Sorry, but users must be at least 13 years of age to register. ";
// the < operator is the "less than" operator for PHP.
}
else {
processRegistration (); // runs a function that processes the user registration.
// This only runs if the user is 13 or over. If the user was under 13, the IF block above would have run instead of this block of code. Remember, in no circumstance will the "ELSE" block ever run if the IF block has run.
}
If you would like to play around with these scripts, copy and paste the code below and give it a try. 
Code: <?php
// play around with the following variable
$age = 12;
if ($age < 13) {
echo "Sorry, but users must be at least 13 years of age to register. ";
// the < operator is the "less than" operator for PHP.
}
else {
processRegistration (); // runs a function that processes the user registration.
// This only runs if the user is 13 or over. If the user was under 13, the IF block above would have run instead of this block of code. Remember, in no circumstance will the "ELSE" block ever run if the IF block has run.
}
?>
In the next tutorial, we will explore how HTML forms are set up and how we can design the first interactive applications. The fun stuff will be beginning soon. 
Stay tuned!
Next Tutorial: Let the fun stuff begin; forms and PHP introduction
Directory of PHP Tutorials
|
|
|
What are your goals |
Posted by: Tyler - August 13th, 2013 at 5:19 AM - Forum: General Discussion
- Replies (3)
|
 |
What are your goals for your websites, forums, blogs ect?
I'd love to hit 50 members and than 100, I think 100 people is still a good amount even though some of these forums hit thousands, I'd be happy with 100. I'd also like to hit 1,000 posts. (my goals for now) if my forums is successful I'd love to hit 1,000 members and 100k posts. I'd also like to learn more on how to make my forums better (as this was my first time making a forums)
|
|
|
PHP - Part 3 |
Posted by: Darth-Apple - August 13th, 2013 at 12:38 AM - Forum: Software
- No Replies
|
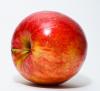 |
How to Program PHP
Tutorial, Part 3
Welcome to part 3 in this PHP tutorial! So far, we've explored basic concepts such as echo statements, and in part 2 we explored variables and gave a brief introduction to arrays. In this tuturial, we'll introduce yet another very fundamental concept in programming. Luckily, this isn't as complicated as it could be in PHP. This tutorial will introduce the concept of the function.
Chances are, you remember functions from Algebra 1. It's probably a term you are already familiar with, but what do functions actually do?
Well, suppose that in your program, you've written about 200 lines of code to verify a file, then upload it to a server. While you are originally writing this code, it is only needed in one part of the program, but as you continue to develop your software, you find out that this code that you have developed is extremely useful for many tasks in your program. Do you simply copy/paste or rewrite the code? You could do that, but it would turn into a massive mess of thousands of lines of code very quickly.
Furthermore, suppose that you find a massive security hole in your code and need to repair it immediately to protect very important data. If you have your code copied several times all throughout your software, you have to fix the hole several times, making it a difficult fix. What's the solution to the delima? A function, of course! A function allows code to be reused.
In PHP, you first must define a function. When you define a function, you give it a name and put some code inside that will be executed. In PHP, a function will only be executed when it is "called". We'll see how this works through some examples.
Code: <?php
function sendGreeting () {
echo "hello world! <br>";
}
?>
This code is pretty much useless. It's not like we'll be reusing the "hello world" echo statement a whole lot in any real projects, but this code is valid code, and it does define a function. If you run this script on your own, you will notice that nothing happens. Why isn't "hello world" appearing on your screen?
In the code above, we defined the function, but we never "called" it. PHP will never execute a function before it is "called".
Code: <?php
function sendGreeting() {
echo "hello world! <br>";
}
sendGreeting();
?>
The only difference in the code above from our original code is the sendGreeting(); line. This line "calls" the sendGreeting function that we defined before. Run this code in your browser, and you will notice that "hello world!" now appears on your screen.
Code: <?php
function sendGreeting() {
echo "hello world! <br>";
}
sendGreeting();
sendGreeting();
sendGreeting();
sendGreeting();
?>
You probably notice that we are calling the sendGreeting function multiple times in the code above. This is perfectly legal in PHP. The really cool thing about functions is that it basically allows you to reuse code anywhere in your program without having to rewrite it. That's what makes it possible to send you four greetings without four echo statements.
Functions can do a lot more than just send greetings. It turns out that every function can take paremeters, and it can return a value. Now, before you get confused about peremeters and return values...
A paremeter is: just a variable that you send to the function.
A return value is: Just a variable that the function sends back to the script that had called the function.
It turns out that both concepts are incredibly useful. Suppose we want to have two kinds of greetings, but we want to save code and define only one function. Well, since we can pass paremeters to the function, we can pass a number to define which greeting to use.
Code: <?php
function sendGretting($selector) {
if ($selector == 1) {
echo "hello world! <br>";
}
else {
echo "why hello there! This is greeting number 2!<br>";
}
return "success printing greeting!";
}
$status = sendGreeting(1);
echo $status;
?>
First, you may notice something new. I haven't talked about the "if" and the "else" statements before. I won't attempt to explain what these do in detail until later tuturials, but these are basically conditionals that only execute code under certain conditions. Basically, if the $selector variable is equal to 1, it will send the first greeting. If it is not equal to 1, it will echo greeting 2 instead. In this code you will see
Quote:hello world!
success printing greeting!
On your screen when you execute this script.
So... what about the "success printing greeting!" Where did that come from? Is "return" some kind of echo statement?
A return value is just some variable that the function sends back once it is done executing. In this case, we just return "success printing greeting!" When we call the function, notice that we have the $status = in front of the actual function. Basically, whatever the function returns will get stored in the $status variable. Because the function returned "success printing greeting!", $status contains "success printing greeting!" and when we echo $status, you see "success printing greeting!" on your screen.
The function is an incredibly useful concept in programming. In the next tutorials, I will further introduce conditionals, which will be a very useful concept later on.
Next Tutorial: Conditional Statements
Directory of PHP Tutorials
|
|
|
PHP - Part 2 |
Posted by: Darth-Apple - August 13th, 2013 at 12:37 AM - Forum: Software
- Replies (2)
|
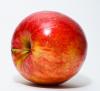 |
How to Program PHP
Tutorial, Part 2
Welcome to part 2 in this PHP tutorial tutorial! In the previous tutorial, we explored the basic "echo" statement, and some pretty fundamental concepts in PHP statements, such as the semicolon at the end of every statement. In this tutorial, we'll explore variables, which are a pretty fundamental concept that we'll be using later on for interactive applications.
When many people see "variables" in the context of computer programming, algebra quickly comes to mind. Don't worry, you won't need to brush up on your mathematical equations. Variables are a simple concept in programming. 
What is a variable?
A variable is essentially just a container that has a value. Yes, these variables have real world applications in algebra, but we won't be getting into sines, cosines, quadratic equations, derivatives, or anything else of that sort. As far as we're concerned, a variable simply holds a value for later use.
Variables in PHP are destroyed as soon as the script is finished executing, so they don't store data long term. If you need to process factory orders or user registrations, a database will be required for that. Nevertheless, variables are very much an important concept that will be foundational to every PHP application. 
Code: <?php
$var1 = 5;
$var2 = 9;
echo "var1 + var2: ";
$result = $var1 + $var2;
echo $result;
?>
Following yet? Yes, that looks like giberish. 
Variables always start with a dollar sign in PHP ($), so you should be able to recognize where the variables are just by looking at the above code. As you can see, in the first two lines of this code, we simply define $var1 to be 5, and $var2 to be 9. These are the values that these variables contain. We could have named these variables anything. We did not have to name then $var1, $var2, etc... We could have called them $apple, $banana, etc... and they would have functioned exactly the same way.
The next statement is just an echo statement. You may be tempted to think that this first echo statement will add var1 and var2, sending 14 to the browser for you to see on the page, but remember that because the text is in quotes, this is just a text string. Since we're only sending a text string, you will literally see "var1 + var2: " in the browser from this statement.
The next statement adds $var1 and $var2, then puts the answer into the $result variable. Remember, we defined $var1 to be equal to 5, and $var2 to be equal to 9. When we add them, they will equal 14, and that answer goes into $result. As stated before, these variables could have been named anything. It's always wise to keep variable names as descriptive as possible in programming, as it does help a lot when you start writing larger scripts confidently. To be honest, $var1 and $var2 aren't the most descriptive names, but you see the idea.
The next statement echoes $result, which of course equals 14 and is displayed in the browser. I encourage you to try this script yourself on your own server or WAMP. Try playing around with it some to get a feel for it. Variables are an important concept in PHP, and they are very simple once you get the hang of it.
More examples
There is quite a lot that is possible with variables, well beyond simply adding numbers. Here is another example. I encourage you to try this script out on your own, playing around with the different numbers and math operators to get a feel for how it works.
Code: <?php
$apples = 5;
$oranges = 995;
$cucumbers = 3;
$result = $apples + $oranges + $cucumbers;
echo "total fruits: ";
echo $result;
echo "<br>"; // the <br> tag is the HTML tag for a new line.
$good_fruits = $result - $cucumbers; // we don't like cucumbers.
echo "Since we don't like cucumbers, the number of good fruits is: ";
echo $good_fruits;
?>
As you can see, we've played around with the math in these statements, using some addition and subtraction. I encourage you to try these scripts out on your own to get a feel for how variables work in use.
Text in variables
In algebra, variables always contained numbers. In programming, variables can contain practically anything. Some kinds of variables (which we'll get to later) can even contain files. Often, you'll see variables with text in them. PHP deals with text variables the same way that it deals with variables containing numbers. (Just don't try to perform a "text string" + "text string #2" addition operation. I've never really tried that to see what exactly PHP says about that, but I'm sure you will get some sort of complaint about attempting a math operation on text. )
Code: $mytext = "this is a text string inside of a variable";
Arrays
So that's more or less the basics, but there is more to variables that needs to be said. An array is a concept we'll be using very shortly that makes it very simple to organize data if you have a lot of data to organize. Essentially, an array is a variable that contains more than one value. Here is how arrays look in code:
Code: <?php
$fruits['apples'] = 5;
$fruits['oranges'] = 9;
$total_fruits = $fruits['apples'] + $fruits['oranges'];
echo $total_fruits;
?>
As you can see, both the number of apples and the number of oranges are stored into the "fruits" variable, but we've made use of an array. In an array, each individual "value" that it stores is called an element. Here, 'oranges' and 'apples' are both elements of the array that is called $fruits. $fruits['oranges'] = 9 is a statement that sets the "oranges" element in the array to 9. It won't affect the "apples" element since "apples" is in a different part of the array. Also, notice how 'oranges' and 'apples' are in brackets. Arrays always have to structured like this so that PHP knows that you are referring to an array in code. Arrays may not seem necessary in this particular situation, but they will become very useful later.
It turns out arrays don't just have to have text in the brackets. We could have used numbers as well.
Code: <?php
$fruits[1] = 5;
$fruits[2] = 9;
$total_fruits = $fruits[1] + $fruits[2];
echo $total_fruits;
?>
The code above functions exactly the same way as the original arrays example does. If you run this script in your browser, you will see the exact same result. The only difference is that we identified the different "elements" of the $fruits array with numbers instead of with text strings. Either way is valid in PHP, although for this particular example, it is much more practical for us to use text strings instead, for obvious reasons. 
Arrays can be incredibly useful when it comes to some applications. Two variables, to be specific, are very important to dealing with HTML forms. We'll be dealing with $_GET and $_POST arrays later on, but these are basically arrays that contain the values of URL fields and form fields, and we'll be getting to those later on.
The next tutorial...
In the next tutorial, we'll be building on more concepts putting these variables to use. We'll introduce the function. stay tuned!
Next Tutorial: Introduction to Functions
Directory of PHP Tutorials
|
|
|
|