Welcome, Guest |
Welcome to Makestation! We are a creative arts/indie discussion community — Your center for creative arts discussion, unleashed!
Please note that you must log in to participate in discussions on the forum. If you do not have an account, you can create one here. We hope you enjoy the forum!
|
|
Update your status...
|
|
Thomas
October 24th, 2024
Update your status...
|
|
Selena98
October 19th, 2024
Update your status...
|
|
Thomas
September 18th, 2024
what a d*** shame
|
|
writingsharks
August 16th, 2024
Writing Sharks is a team of professional writers that provides 100% original, high-quality, plagiarism-free content. Our services include essay writing, research paper writing, term paper writing, academic writing help, and much more. Visit today to order your writing.
https://writingsharks.net/
|
View all updates
|
Online Users |
There are currently 335 online users. » 0 Member(s) | 333 Guest(s) Baidu, Google
|
Latest Threads |
Why Should You Use a Home...
Forum: Announcements
Last Post: arhanshaikh
November 26th, 2024 at 9:56 AM
» Replies: 1
» Views: 161
|
Forum game, I'm traveling...
Forum: Forum Games
Last Post: novavoyager
November 18th, 2024 at 9:16 PM
» Replies: 11
» Views: 7,473
|
Buy Bank Logs with email ...
Forum: Media & Entertainment
Last Post: MrVenus
November 14th, 2024 at 8:02 PM
» Replies: 0
» Views: 115
|
Buy Bank Logs with email ...
Forum: Web Design & Internet
Last Post: MrVenus
November 14th, 2024 at 8:01 PM
» Replies: 0
» Views: 98
|
Buy Bank Logs with email ...
Forum: General Discussion
Last Post: MrVenus
November 14th, 2024 at 8:01 PM
» Replies: 0
» Views: 105
|
Oh big steve.
Forum: Forum Games
Last Post: alex888
November 14th, 2024 at 5:44 PM
» Replies: 5
» Views: 3,416
|
Guess the movie(s) in 5 w...
Forum: Forum Games
Last Post: alex888
November 14th, 2024 at 2:25 PM
» Replies: 9
» Views: 11,352
|
|
|
TV Time |
Posted by: campingrhino - June 1st, 2020 at 1:21 PM - Forum: Media & Entertainment
- Replies (4)
|
 |
Has anyone used this?
TV Time
I find it quite useful for keeping track of the never-ending selection of shows I've started but not finished and it's great for notifying you of when a series you watched years ago has new episodes (when it actually syncs with TVDb that is).
|
|
|
Pipes, Forks, and IPC |
Posted by: Lain - June 1st, 2020 at 1:52 AM - Forum: Technology & Hardware
- Replies (4)
|
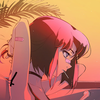 |
So recently, the lady started her Summer semester and has an Operating Systems class going on now.
The professor said they need to use C for the class. The problem is that the computer science faculty doesn't actually TEACH C until like fourth year right before you get your bachelor's, for some reason. Now the professor said that only the first assignment needs to be done in C and the rest can be done in Java.
How you'd do any low-level work with Java is completely beyond me, you can't even pass by reference in the language, let alone fork a process.
But in any case, she knows that C is my favourite language, and as a result, she came running to me when her code wouldn't compile and she needed help getting started with gcc anyway (For Windows, so naturally it would be too f*** for a complete novice to figure out.)
The Assignment and Setup:
The assignment seems really simple. Only a few lines of code. My solution did it in 75 including some whitespace and extra lines for opening curly braces.
In a (very) watered down summary:
Quote:Write a C program that uses fork() to create new processes, and make those processes write data to a pipe, and read that data from the pipe.
Now I didn't read the whole assignment, so I was missing specifics, but her code was really messy (I don't blame her, this was her first C program) and I decided to do a full rewrite.
The first thing I told her after hearing about the assignment and looking at her code is that it would be impossible to do on Windows, since that's what she was trying to do. The main problem lies in the fact that fork() isn't supported on Windows to create new processes. The other problem is that this was asking to use a variety of Linux syscalls that also aren't implemented quite the same way on Windows.
So instead, I ran her through installing Debian under the Windows Subsystem for Linux (WSL) since she has used Debian in the past and was relatively comfortable with it. Then we installed gcc and made sure it was all working correctly. finally, she got WSL setup to be the main command-line in visual Studio Code (the lightweight FOSS text-editor IDE) so she could do all the development in one place.
More Specifics and How I Cheated:
The only familiarity I have with Linux syscalls is with assembly, and using those calls is very different than using them in C. So as a result, there was a pretty big learning curve for me as well
The professor included a main() that would call the desired function so that wouldn't need to be written. It's a messy snippet, but acceptable enough for the task at hand.
I got hold of that main() and started to get to work.
Here's the rough pseudocode that she had so you can get an idea of functionality:
Code: int main() {
functionCallForForking(n);
return 0;
}
void functionCallForForking(int n) {
if(n == 1) {
write(Process 1 Started);
write(Process 1 Ended);
exit();
}
int forks = fork();
if(child) {
write(Process n Started);
functionCallForForking(n-1);
write(Process n Ended);
} else if(parent) {
wait();
exit();
} else {
printf(fork failed);
exit()
}
}
Extremely simplified. It was more like 100 lines.
But you can see the pattern. It forks, if it's the child process, it'll fork again, and if it's the parent, it'll wait for the child process to finish.
It should iterate over n processes to start, given by the command line argument which I omitted for simplicity.
Output:
Code: $ ./a.out 5
Process 5 Started
Process 4 Started
Process 3 Started
Process 2 Started
Process 1 Started
Process 1 Ended
Process 2 Ended
Process 3 Ended
Process 4 Ended
Process 5 Ended
$ _
Now, this needs to use a Pipe structure, which is basically a queue structure, which is to say that the first thing to go into the pipe is the first thing to come out of the pipe. Think of it as a line-up at your grocery store. Whoever gets there first gets their purchase processed first, and they are the first to leave.
The tricky part is that the pipe is defined as a file descriptor.
On Linux, files can only really be accessed by a single process at the same time for writing. If you don't then you get race conditions. More on this later.
But the point is that because we're forking multiple processes that are all writing to the same pipe (or a virtual file in memory, rather,) then we're going to need to make sure that our processes do not interfere with each other during their respective reading and writing.
So here's how I cheated:
I only allowed the root process (Process 5 in the example above) to read from the pipe.
Then I changed when each process writes to the pipe.
|
|
|
Hello! |
Posted by: campingrhino - June 1st, 2020 at 12:38 AM - Forum: Introductions
- Replies (6)
|
 |
Hi everyone!
My name's Ryan, I'm from the UK and I love making things and I thought I'd join. Saw this website on the MyBB forums and thought it's a community for me.
I make websites and web apps, occasionally games and I've just launched a web development forum (part of why I was lurking on the MyBB forums!) and I'm happy to be here.
|
|
|
Clint Eastwood: Hollywood's paradoxical superstar turns 90 |
Posted by: tc4me - May 31st, 2020 at 6:59 PM - Forum: Media & Entertainment
- No Replies
|
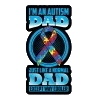 |
Clint Eastwood: Hollywood's paradoxical superstar turns 90 A spaghetti western star whose 'Dirty Harry' films made him an anti-hero of the political right, the Oscar-winning actor and director later praised Donald Trump. But as he turns 90, his true legacy is as an auteur.
|
|
|
After nine years, the United States flew into space |
Posted by: tc4me - May 31st, 2020 at 4:23 PM - Forum: Current Events
- Replies (2)
|
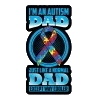 |
After nine years, the United States flew into space again on its own and the journey of the two astronauts Bob Behnken and Douglas Hurley is already over. Because on Sunday afternoon, the Crew-Dragon space capsule from SpaceX docked on schedule with the international space station ISS. NASA chief Jim Bridenstine wrote on Twitter: "Welcome home!" Congratulations came from Russia. Roskosmos boss Dmitri Rogosin wrote to his US colleague Bridenstine on Twitter: "Bravo!"
|
|
|
Member of the Month VOTE - June 2020 |
Posted by: Guardian - May 31st, 2020 at 3:26 PM - Forum: Community Related
- No Replies
|
 |
Please make your selection or the Member of the Month for June 2020.
This vote is to recognize members for their contributions for the previous month. In this case, a user's contributions for the month of May lead to their nomination for the June Member of the Month.
The poll is anonymous, and no one will see your vote.
Member of the Month candidates:
Altair
s3_gunzel
tc4me
|
|
|
Stack Overflow |
Posted by: Darth-Apple - May 31st, 2020 at 4:56 AM - Forum: Software
- Replies (18)
|
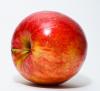 |
In my limited experience, they are some incredibly rude people. At first glance, it seems like a great place, and I've found great answers there. They take quality very seriously (which I respect). But my experiences haven't been particularly good.
They have a lot of rules (not a bad thing), but they are sort of open to interpretation and they are really only worthwhile if you have an extremely precise problem. It's not an ordinary forum where you can ask more generalized questions to get feedback, information on best practices, or otherwise. It's more of a "here is my code, here is my problem, how do I do X."
If you're newer to the community, it's really more up to the discretion of whoever sees the problem as to what ends up being done, and often something gets downvoted only because it competes with someone else's question or answer. Everyone seems to be in a repuation war almost constantly.
Is there anyone here who has successfully "figured out" stack overflow? If so, how do you go about participating meaningfully?
|
|
|
Drinks near a laptop, etc |
Posted by: tc4me - May 29th, 2020 at 7:42 AM - Forum: The Others
- Replies (6)
|
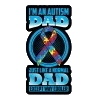 |
Hello good morning, are you also like me and put your coffee cup next to laptop printer cell phone etc?
Today it was time again, a expensive coffee, because I acted carelessly, spilled the coffee, via printer (Brother MFC-L3750CDW) 500Euro, and it no longer works, moreover via the power bar, charging plug from my Samsung Indiktiv, charger, the plug also no longer works, makes a short circuit, fuses Fi in the house fall out. I'm such a jerk ... annoy me
|
|
|
|