Welcome, Guest |
Welcome to Makestation! We are a creative arts/indie discussion community — Your center for creative arts discussion, unleashed!
Please note that you must log in to participate in discussions on the forum. If you do not have an account, you can create one here. We hope you enjoy the forum!
|
Forum Statistics |
» Members: 958
» Latest member: Aruz258
» Forum threads: 4,636
» Forum posts: 28,884
Full Statistics
|
|
|
PHP - Part 1 |
Posted by: Darth-Apple - August 13th, 2013 at 12:36 AM - Forum: Software
- Replies (4)
|
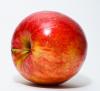 |
How to Program PHP
Tutorial, Part 1
Ever wanted to get your feet wet with a programming language, but simply haven't had the money to pay for expensive books or online courses? Of course, any programming language takes time to master, but I will attempt to cover the introduction to the PHP programming language through these tutorials. I'm living proof that you don't need an $80 manual to learn a programming language. Due to the fact that I can't write 400 pages worth of really awesome content effectively without writing a book, I won't attempt to dive into concepts that are too deep, but I hope I'll be able to give you a good introduction to the language. If you'd like to go further, w3schools is a great reference resource that I've used, and once you've gotten your feet wet, it is a great resource to use if you want to continue past these tutorials.
I'm assuming that anyone reading this already has a good understanding of basic HTML, and at least knows what they are looking at if they see some javascript. If you are not at this stage, I won't attempt to review any HTML in these tutorials, so I'd recommend taking a good HTML course first. I would personally recommend codeacademy.com if you are completely new to HTML, but if HTML is not new to you, then we're ready to dive in!
PHP introduction - part 1
What exactly is PHP anyway?
PHP is a programming language that runs straight from the server, and is used to power many websites. Forum software, social networking solutions, or any other software solution that works with dynamic content will usually use PHP (or a similar language) to generate a page, then will send it to the browser. PHP is extremely useful for that reason. It differs from HTML because it is not a markup language that simply defines the format that a page is sent to the browser with. PHP is a programming language that can be used to generate the HTML pages sent to the browser.
Of course, there are alternatives as well. PHP is probably the most common language used to achieve its task (for non enterprise use, at least), so this will be the first tutorial I will write here at Makestation.
Hold on, prep work first! ( skip this section if you already have a server or localhost )
While you certainly might be able to get familiar with some concepts you haven't seen before by simply reading these tutorials, you are much more likely to get the hang of any programming language by actually following along with the tutorials with your own scripts. You do not need to have a server to write and execute PHP scripts. For windows users, I recommend installing wampserver to your computer, which will allow you to run PHP files straight from your computer. If you cannot get wampserver to work, a simple free webhost such as zymic or 000webhost should be enough for this project. (Be sure to read your webhost's terms of service carefully if you choose to go that route. )
I would strongly recommend setting this up before continuing so that you can follow along with these tutorials with your own scripts. If you are a windows user, WAMP is probably the easiest route. Once you have that set up, simply copy/paste the scripts that are posted here into a .php file inside of the c:/wamp/www directory, and as long as wampserver is started on your computer, you can go to localhost/myfilename.php in the browser of your choice to execute it.
Your first PHP script
I've seen quite a few tutorials that spend quite a bit of time teaching the theory behind a concept before actually jumping to the concept itself. Personally, I'm the kind of guy who likes to just jump straight into something and see stuff happen, so I won't be wasting too much time. In these tutorials, I will try to give a brief introuction, go straight to the code, then explain it afterwards. If something looks a little confusing, don't worry. Just keep reading, and I'll explain it later on. So, without wasting time, here will be your first PHP script.
Code: <?php
echo "this is my first php script!";
?>
The first thing that is important are the <?php an the ?> tags. Simply naming a file .php isn't enough for PHP to actually execute the code as PHP. Without the <?php and ?> tags, the PHP server will assume that your file is actually an HTML file that is simply named as PHP file. In other words, all PHP code must be enclosed in the opening and closing PHP tags in order for it to actually be executed by the server.
The echo command is the command that sends data to the browser, which in this case is the "this is your first php script" message. This command is probably the most important command in PHP, since it's what sends data generated by the PHP script to the browser. (As stated before, no PHP code actually gets sent to your browser. PHP is all processed on the server, so all that the browser will ultimately receive is an HTML page generated and sent by the PHP script. )
Also, notice the semicolon (the ; character) at the end of the line. This character must go at the end of every statement in PHP. It essentially tells PHP that this command is complete, and that it is ready to execute. If the semicolon is missing, PHP will get confused and flag an error instead of executing the PHP code. It turns out that a missing semicolon is one of the most common errors in code that PHP programmers make. Whenever I see an error in my code, the first thing I'll usually check for is a missing semicolon.
Example 2:
Code: <?php
echo "this is line #1";
echo "this is line #2";
echo "Why are you still reading this? ";
echo "forget it! PHP is easy! ";
?>
As you can see, we've made use of more echo statements. Each one has a semicolon at the end of it to mark the end of the command. If the semicolon is removed, PHP will get confused as it executes the code because it won't know where the end of the statement is. Also, notice again how all code is inside of the <?php and ?> tags.
Introduction to PHP - part 1.5
We've just gotten into the absolute basics of how a PHP file could be structured, what we need to do in order to get it to execute, and how to send text to the browser, but there are a few other quick concepts we'll need to dive into before we enter the next tutorials. We'll be getting into more interesting concepts in the coming tutorials, but for now we need to make sure we understand what we need to know before moving on.
Anyway, the first concept we'll get into before heading into the next tutorials is the concept of the text string. This is really more a definition we'll be bringing up later. A text string is exactly what it sounds like. It's just a string of text. For example, for this line of code...
Code: <?php
echo "hello world!";
?>
"hello world" is just a text string. Now there is nothing complicated about that, but what if we need to merge two strings into one string?
Code: <?php
echo "string 1 is " . "going to be combined with string 2";
?>
Try running this script, and you will see "string 1 is going to be combined with string 2". Notice how the period between the two strings combined both strings into one. This is one of many "operators" in PHP that can manipulate data in different ways. PHP also has many additional operators. The simplest of these operators are the math operators (+, -, and so on... ) We'll get into more of these operators in the later tutorials. For now, I encourage you to try these scripts on your own to ensure you understand these concepts.
Either way, we'll probably be using the period to combine different strings quite frequently in later tutorials, so feel free to play around with the above example to get a feel for how it works.
PHP comments
In PHP, it is possible to make notes in the code without it actually executing as code. There are multiple formats that you can use to define any text in the code as a comment, but for these tutorials, we'll just put "//" at the beginning of the line. This tells PHP that the rest of the line is not code, so it will just skip over it. This is very useful for making notes to yourself about the code.
Code: <?php
echo "hello world!";
// this is a comment! It will not execute, since we have two slashes at the beginning of the line.
?>
The next tutorial
In the next tutorial, we'll begin to explore variables. Once we get some basic concepts down in the next few tutorials, we can begin playing around with some interactive applications. Stay tuned! 
Next Tutorial: Introduction to Variables
- Directory of PHP Tutorials
|
|
|
Comet Sighted |
Posted by: Acko - August 12th, 2013 at 3:15 AM - Forum: Forum Games
- Replies (2)
|
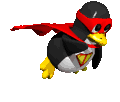 |
Peasants are always superstitious, and the appearance of a comet in the sky has caused panic among our people. They are convinced that this is a sign that the end of times is near or that something bad is going to happen in the near future.
|
|
|
Must have MyBB plugins |
Posted by: Darth-Apple - August 11th, 2013 at 9:30 PM - Forum: MyBB Related
- Replies (2)
|
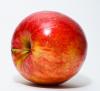 |
MyBB is certainly not bad software out of the box, but undoubtedly one of its biggest advantages is its extensibility and its great plugin/template system. Installing and removing plugins, and even writing them, is very easy to do. However, although there are certainly many plugins out on the market today, it can be hard to pinpoint the some of the popular plugins used by forums today. This is certainly not intended as an all-inclusive list, but I figured I'd post this for people looking for some good ideas for plugins to use on their forum.
MyAlerts
This plugin adds a fully functioning xenforo-style alerts system for MyBB, and works quite well. It's worth considering for any MyBB forum, and the latest version is pretty stable. Just make sure to use github to download the mod. The author, due to the fact that the official MyBB mod site is extremely buggy, refuses to update the mod on the MyBB mod site. In fact, I don't blame him. I have never even been able to get a file to even upload, much less get a mod approved!
Page Manager
This is a very simple and effective mod for adding an unlimited number of additional HTML pages to your MyBB forum. It undoubtedly is a must have for any forum.
Profile Comments
Profile Comments is another one of those simple essentials for MyBB. It allows users to comment on the profiles of other members, and is definitely a very useful plugin for any MyBB forum.
Registration Security Question
This is a great plugin that will significantly reduce the amount of spam that reaches your forums. Considering it doesn't take much these days for the spambots to find your forum, this is undoubtedly a must-have for all MyBB users to protect their forums from getting mega-spammed by spambots.
Sidebox
This is a pretty simple mod that allows you to put portal widgets on a sidebar for your forum. This may not necessarily work for all themes, but if you're looking for a way to get the latest threads list on the sidebar of your forum, this plugin is for you.
NewPoints
Newpoints is a fairly popular points and "currency" management plugin that is used for a variety of different purposes. It is highly configurable, and many forums have found it immensely useful.
Thanks
This plugin adds a "thanks" button below every post on the forum. I personally love the plugin. It adds a nice element to posting on the forums, and it's a nice way to "thank" someone for a post as an alternative to the +rep feature.
Someimage Image Uploader
This plugin adds a simple image upload form on the new reply and new post pages, allowing users to quickly and easily upload images in the post editor. It also allows users to select sizes for thumbnails and automatically creates the BBcode for them. For users who aren't well accustomed to using an image host, this plugin can be a nice addition to any forum.
Of course there is quite a large selection of MyBB plugins out there, and quite a few of them are definitely worth trying. If you have any other suggestions for the list, feel free to add them below.
|
|
|
Makestation - theme update #1 |
Posted by: Darth-Apple - August 11th, 2013 at 7:42 PM - Forum: Announcements
- Replies (7)
|
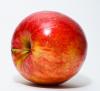 |
As most of you know, Makestation is running a custom theme exclusive to Makestation. The original theme received quite a bit of feedback, but there were a few things that weren't really ever finished in the original development. I'm now pleased to say that an updated version of the theme is now installed. I think you'll notice the difference. It includes...
* CSS buttons. Most buttons have now been converted. The remaining buttons will be converted in a future update.
* Improved postbit designs.
* A sidebar, with a new style for portal boxes and a new page layout.
* An alerts plugin that gives Makestation a xenforo-like notifications system.
* Some bug fixes.
This is a minor update, and there will also be future updates with more improvements. If you come up with something you'd like to see in a future update, or notice something that is fishy or out of place, let us know here!
UPDATE: We will be doing another very minor theme update in the next couple of days to fix a few additional bugs. If you notice a glitch or something that looks out of place, please don't hesitate to let us know!
|
|
|
someimage.com - a good alternative to photobucket |
Posted by: Darth-Apple - August 10th, 2013 at 7:06 PM - Forum: Web Design & Internet
- Replies (1)
|
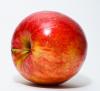 |
Personally, I've always felt that the ridiculous bandwidth/image size restrictions, photo albums, unneeded features, and of course the ads, were quite annoying with photobucket, imageshack, etc...
Someimage.com is a pretty neat imagehost for people who just need a simple image hosting service, and it doesn't have a bunch of unnecessary clutter that isn't useful. It is also owned by the owner of icyboards, which is a growing forum host with a great reputation.
Anyway, someimage is worth checking out if you haven't checked it out already. It's worth a look.
|
|
|
|